To get the value on a display we first need to crop the display in the image.
This is an example source image:
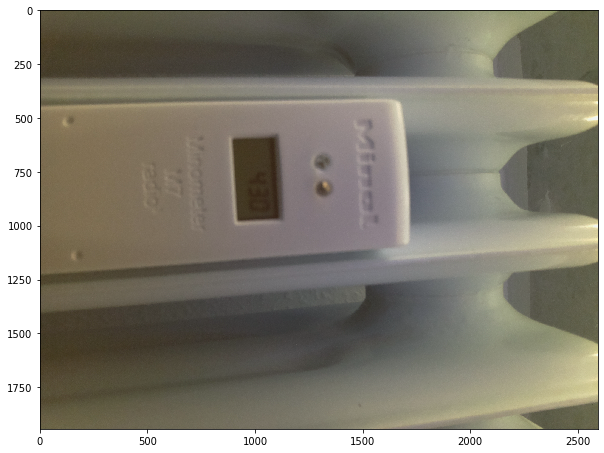
The only Python package used is opencv.
Import OpenCV:
Load image and get middle of image:
image = cv2.imread("../images/1538832301.jpg")
image = image[300:1300, 500:1400]
height, width, channels = image.shape
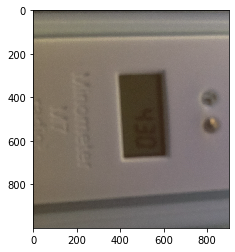
Rotate image:
center = (width / 2, height / 2)
angle = 87
M = cv2.getRotationMatrix2D(center, angle, 1)
image = cv2.warpAffine(image, M, (height, width))
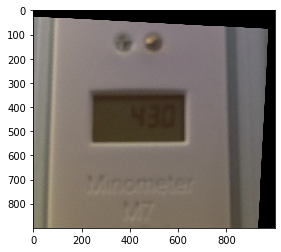
Convert to grayscale:
gray = cv2.cvtColor(image.copy(), cv2.COLOR_BGR2GRAY)
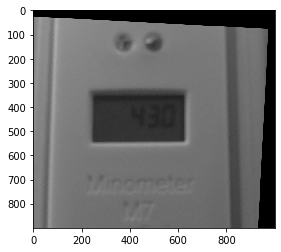
Blur and threshold:
gray = cv2.blur(gray, (11, 11))
thresh = cv2.threshold(gray, 60, 255, cv2.THRESH_BINARY)[1]
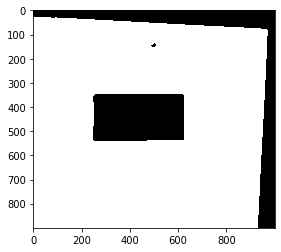
Find contour of box in the middle of the image and plot in green on image.
The box has a minimum of 100 pixel width and a minimum of 50 pixel height.
contours,hierarchy = cv2.findContours(thresh, 1, 2)
for cnt in contours:
x,y,w,h = cv2.boundingRect(cnt)
if w>100 and h>50:
break
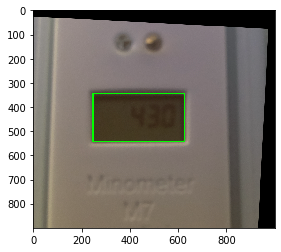
Now crop image based on the points of the green box:
cropped = image[y:y+h, x:x+w]
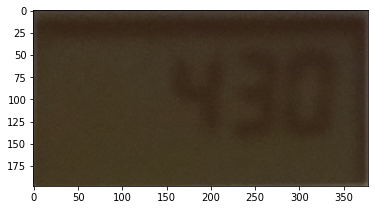
Increase alpha contrast of the image to see the digits and save result to disk:
contrast = cv2.convertScaleAbs(cropped, alpha=3, beta=0)
cv2.imwrite('cropped_image.png', cropped)
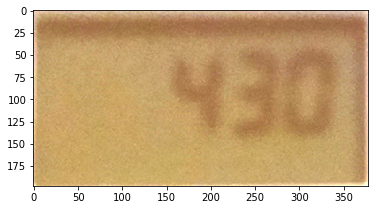
The next step will be to recognize the numbers in the display.