Draw Geo Points into a Shape
How to draw points on a shapefile? As an example we will do this with Germany and draw a few example cities with the correct location into the shape.
First download the shapefile for Germany from arcgis and unpack the zip-file. The file needed here is "LAN_ew_21.shp". There are of course a lot of different possibilities to get a shp file or a GeoJson version of the state borders of Germany. Feel free to try others.
The following code plots a few cities onto the Germany shape by using geopandas and matplotlib.
import json from io import StringIO import geopandas import matplotlib.pyplot as plt states = geopandas.read_file("LAN_ew_21.shp") # some random cities as GeoJSON cities = geopandas.read_file( StringIO( json.dumps( { "type": "FeatureCollection", "features": [ { "type": "Feature", "geometry": {"type": "Point", "coordinates": [7.85, 47.995]}, "properties": {"name": "Freiburg im Breisgau"}, }, { "type": "Feature", "geometry": {"type": "Point", "coordinates": [9.738611, 52.374444]}, "properties": {"name": "Hanover"}, }, { "type": "Feature", "geometry": {"type": "Point", "coordinates": [13.74, 51.05]}, "properties": {"name": "Dresden"}, }]}))) # plot shape and cities into same plot fig, ax = plt.subplots() ax.set_axis_off() states.plot(ax=ax, color="white", edgecolor="black") cities.plot(ax=ax, marker="o", color="red", markersize=7) plt.savefig("plot.png", bbox_inches="tight")
The resulting plot:
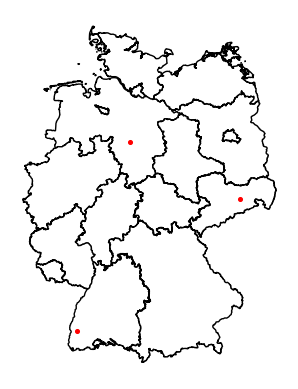